Coding Interview Tips
Tue Jun 28 2022
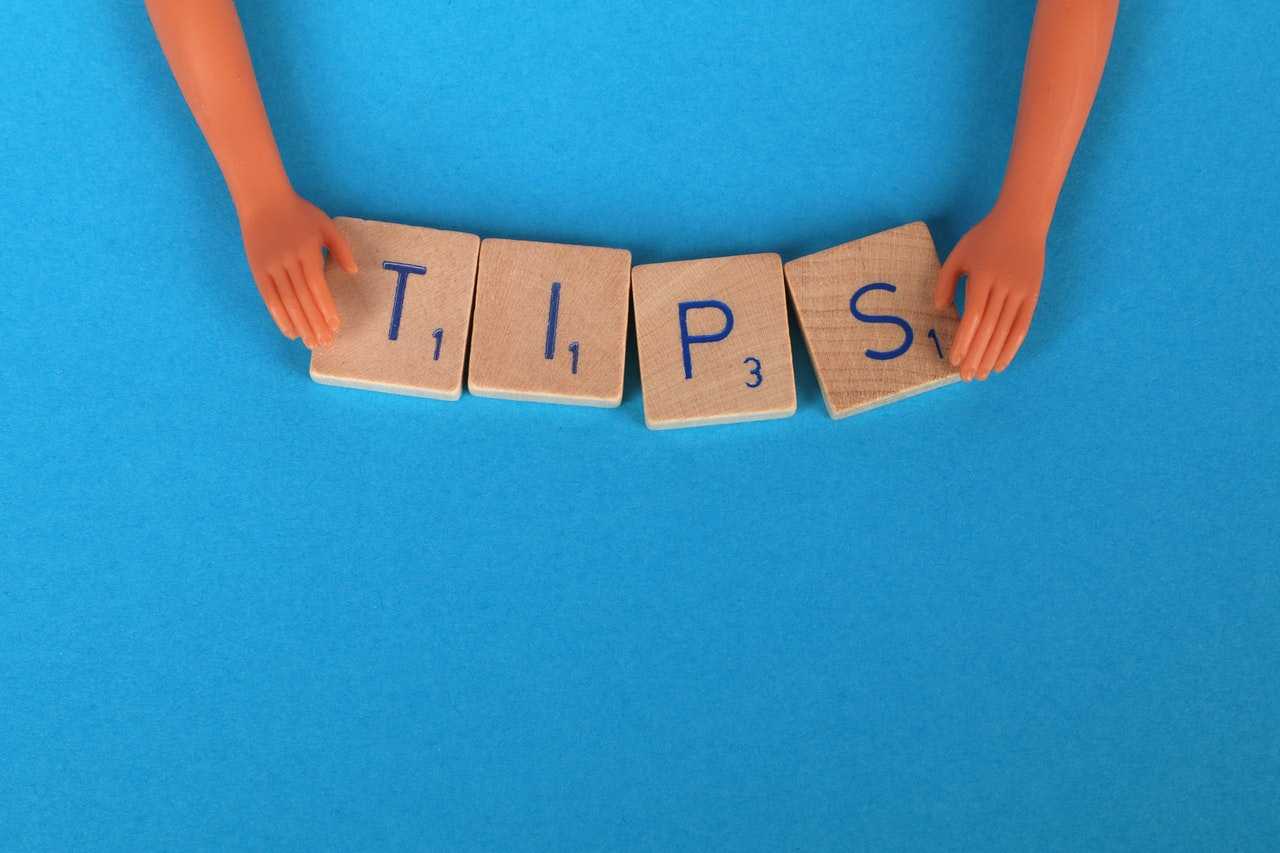
Generate a list of 0,1 as binary representation. It's great if you have any challeges that can use bit manipulations. For example return all permutations of a list.
def createBin(size):
num = 2**size - 1
binned = bin(num)
res = [int(d) for d in str(binned)[2:]]
return res
create linked list from an array, transform linked list to an array:
def buildList(arr):
fake_head = ListNode()
prev = fake_head
for item in arr:
next_val = ListNode(item)
prev.next = next_val
prev = next_val
return fake_head.next
def getArrFromList(head):
arr = []
cur = head
while cur:
arr.append(cur.val)
cur = cur.next
return arr
heap with custom comparator:
class Node(object):
def __init__(self, count, val):
self.count = count
self.val = val
def __lt__(self, other):
return self.count < other.count
# just a nice print
def __repr__(self):
return f'{self.val}: {self.count}'
nodes = [Node(1,1), Node(2,2)]
heapify(nodes)
Create a matrix with width*height:
matrix = [[0 for x in range(w)] for y in range(h)]